- [VB.NET] Hướng dẫn fill dữ liệu từ winform vào Microsoft word
- [VB.NET] Hướng dẫn chọn nhiều dòng trên Datagridview
- GIỚI THIỆU TOOL: DUAL MESSENGER TOOLKIT
- [PHẦN MỀM] Giới thiệu Phần mềm Gmap Extractor
- Hướng Dẫn Đăng Nhập Nhiều Tài Khoản Zalo Trên Máy Tính Cực Kỳ Đơn Giản
- [C#] Chia sẻ source code phần mềm đếm số trang tập tin file PDF
- [C#] Cách Sử Dụng DeviceId trong C# Để Tạo Khóa Cho Ứng Dụng
- [SQLSERVER] Loại bỏ Restricted User trên database MSSQL
- [C#] Hướng dẫn tạo mã QRcode Style trên winform
- [C#] Hướng dẫn sử dụng temp mail service api trên winform
- [C#] Hướng dẫn tạo mã thanh toán VietQR Pay không sử dụng API trên winform
- [C#] Hướng Dẫn Tạo Windows Service Đơn Giản Bằng Topshelf
- [C#] Chia sẻ source code đọc dữ liệu từ Google Sheet trên winform
- [C#] Chia sẻ source code tạo mã QR MOMO đa năng Winform
- [C#] Chia sẻ source code phần mềm lên lịch tự động chạy ứng dụng Scheduler Task Winform
- [Phần mềm] Tải và cài đặt phần mềm Sublime Text 4180 full version
- [C#] Hướng dẫn download file từ Minio Server Winform
- [C#] Hướng dẫn đăng nhập zalo login sử dụng API v4 trên winform
- [SOFTWARE] Phần mềm gởi tin nhắn Zalo Marketing Pro giá rẻ mềm nhất thị trường
- [C#] Việt hóa Text Button trên MessageBox Dialog Winform
[C#] Hướng dẫn sử dụng Microsoft Cognitive Services để nhận dạng hình ảnh
Bài viết hôm nay, mình sẽ hướng dẫn các bạn sử dụng Microsoft Cognitive Services để nhận dạng hình ảnh trong lập trình C#.
[C#] Microsoft Cognitive Services là gì?
Microsoft Cognitive Services bao gồm một bộ các API ứng dụng trí tuệ nhân tạo thông minh, cho phép lập trình viên ở mọi cấp độ từ những bạn sinh viên viết ứng dụng đầu tiên của mình hay những lập trình viên chuyên nghiệp làm việc cho những công ty, tổ chức lớn đều có thể tạo ra được những ứng thông minh hơn một cách dễ dàng.
Các API của Cognitive Services được viết dưới dạng REST API
do vậy lập trình viên có thể tích hợp các API này trên nhiều nền tảng khác nhau như iOS, Android, hay Windows, chỉ cần có kết nối Internet.
Tính đến thời điểm viết bài viết này, Microsoft Cognitive Services bao gồm 21 API được chia thành 5 nhóm: Vision
, Speech
, Language
, Knowledge
và Search
. Hãy cùng tìm hiểu 5 nhóm API này là gì?
Bây giờ mình sẽ demo một ứng dụng sử dụng Microsoft Cognitive Services. Khi import hình ảnh vào thì nhận dạng hình ảnh đó là hình ảnh gì qua API.
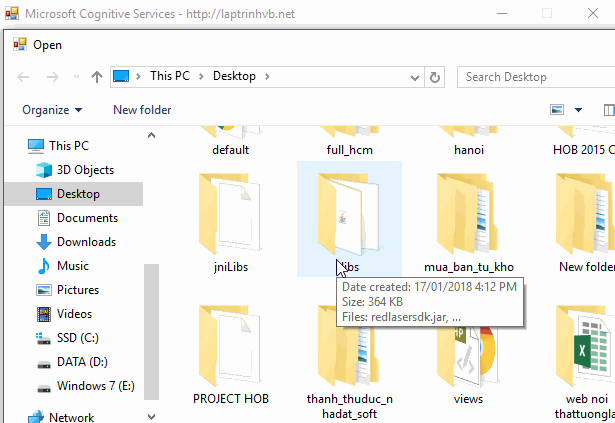
Để sử dụng được Microsoft Cognitive Services, các bạn vào địa chỉ trang web link bên dưới để đăng ký và lấy key API nhé.
Link: https://azure.microsoft.com/en-us/try/cognitive-services/
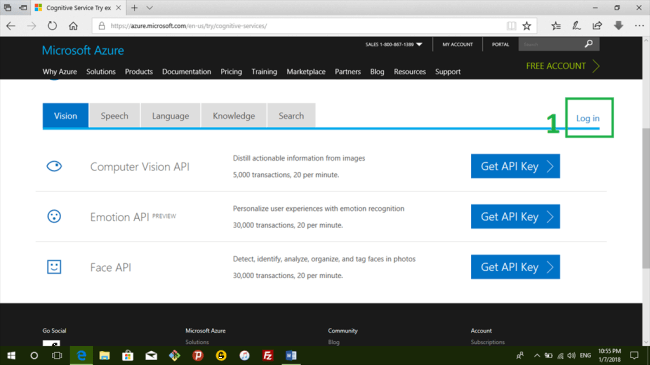
Sau khi, login thành công, các bạn nhấn vào nút Add Vision AP
I để tạo ứng dụng cho mình như hình bên dưới:
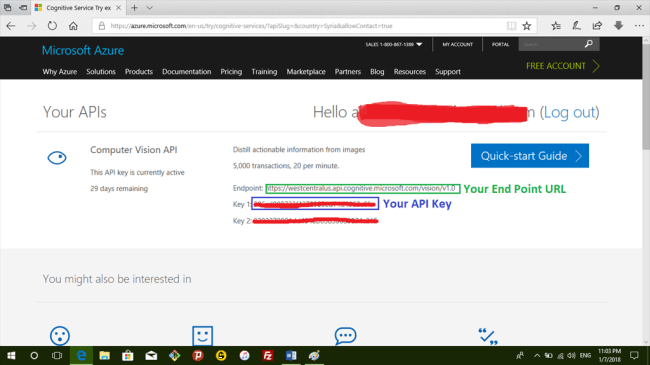
Dưới đây là Source code Microsoft Cognitive Services C#:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Net.Http;
using System.IO;
using Newtonsoft.Json.Linq;
using Newtonsoft.Json;
namespace VisionApi
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
const string subscriptionKey = "386cd088733f4272980ed71d1963c0fa";
const string uriBase = "https://westcentralus.api.cognitive.microsoft.com/vision/v1.0/analyze";
string imageFilePath = "";
private void btnBrowse_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = "JPEG *.jpg|*.jpg|PNG *.png|*.png";
if (ofd.ShowDialog() == DialogResult.OK)
{
imageFilePath = ofd.FileName;
pictureBox1.Image = Image.FromFile(imageFilePath);
btnRecoginze.Enabled = true;
}
}
async Task MakeAnalaysisRequest(string imageFilePath)
{
HttpClient client = new HttpClient();
client.DefaultRequestHeaders.Add("Ocp-Apim-Subscription-Key", subscriptionKey);
string requestParamters = "visualFeatures=Categories,Description,Color&language=en";
string uri = uriBase + "?" + requestParamters;
HttpResponseMessage responseMessage;
byte[] dataByte = GetImageAsBytesArray(imageFilePath);
using (ByteArrayContent content = new ByteArrayContent(dataByte))
{
content.Headers.ContentType = new System.Net.Http.Headers.MediaTypeHeaderValue("application/octet-stream");
responseMessage = await client.PostAsync(uri, content);
string contentString = await responseMessage.Content.ReadAsStringAsync();
return contentString;
}
}
private byte[] GetImageAsBytesArray(string imageFilePath)
{
FileStream fileStream = new FileStream(imageFilePath, FileMode.Open, FileAccess.Read);
BinaryReader reader = new BinaryReader(fileStream);
return reader.ReadBytes((int)fileStream.Length);
}
private async void btnRecoginze_Click(object sender, EventArgs e)
{
btnRecoginze.Enabled = false;
btnBrowse.Enabled = false;
btnRecoginze.Text = "Đang xử lý dữ liệu...";
string result = await MakeAnalaysisRequest(imageFilePath);
if (result == "")
{
label2.Text = "Kết nối thất bại";
}
else
{
JObject data = JObject.Parse(result);
JToken description = data["description"];
string a = (string)data.SelectToken("description.captions[0].text");
label2.Text = a;
}
btnBrowse.Enabled = true;
btnRecoginze.Text = "Bạn thấy gì?";
}
}
}
HAPPY CODING :)